Introduction to C-Language: The Foundation of Modern Programming
C is a powerful and widely-used programming language that has become the foundation of many modern software systems and applications. Originally developed by Dennis Ritchie at Bell Labs in the early 1970s, C was designed for system programming and writing operating systems. Its influence has been vast, giving rise to many other programming languages, including C++, Java, and C#. C is often referred to as a middle-level language because it bridges the gap between high-level programming languages (like Python or Java) and low-level machine languages (like Assembly).
C's simplicity, efficiency, and close relationship to hardware make it an ideal language for understanding the core principles of programming. Learning C helps programmers grasp fundamental concepts such as memory management, data structures, and algorithms, which are crucial for any successful programming career.
Key Features of C
C has several defining characteristics that make it a powerful tool for both system-level and application-level programming:
- Portability: C programs can be written on one system and run on another with little or no modification, making it highly portable across different platforms.
- Efficiency: C offers control over hardware and memory, allowing for optimized and efficient code that can run on limited-resource systems like embedded devices.
- Structured Programming: C supports structured programming principles such as functions, loops, and conditionals, which make programs easier to understand, maintain, and debug.
- Low-Level Manipulation: C allows direct access to memory through pointers, enabling programmers to write low-level code that interacts closely with the hardware.
- Rich Library Support: C has a rich set of libraries that provide functions for various tasks, from basic input/output operations to complex mathematical computations.
Why Learn C?
Learning C offers several advantages to programmers, especially those looking to develop a strong foundation in computer science and software development:
- Foundation for Other Languages: Many modern programming languages, such as C++, Java, and Python, are based on or influenced by C. Mastering C provides a solid foundation for learning these languages more easily.
- System-Level Programming: C is ideal for writing operating systems, embedded systems, and other low-level software that interacts directly with hardware.
- Performance Optimization: C’s ability to manipulate memory and resources at a low level makes it perfect for performance-critical applications where speed and efficiency are paramount.
- Industry Relevance: C is widely used in various industries, including telecommunications, automotive, aerospace, and game development, making it a valuable skill for any programmer.
Applications of C
C is used in a wide range of applications, from system programming to application development. Some common areas where C is used include:
- Operating Systems: Many operating systems, including UNIX, Linux, and parts of Windows, are written in C due to its efficiency and control over system resources.
- Embedded Systems: C is commonly used in programming microcontrollers and embedded systems, where direct hardware interaction and resource management are essential.
- Game Development: Due to its speed and efficiency, C is often used in game engines and performance-critical parts of game development.
- Compilers and Interpreters: Many compilers and interpreters for other programming languages are implemented in C.
Conclusion
Understanding C is fundamental for anyone serious about programming, particularly those interested in system-level programming, embedded systems, or performance-critical applications. The language’s simplicity, portability, and control over system resources make it both a practical tool and an invaluable stepping stone to mastering more advanced programming languages and concepts. As a result, C remains a popular choice for developers and continues to play a key role in modern computing.
Features of C-Language: Why It Remains a Powerhouse in Programming
The C programming language is known for its simplicity, power, and versatility. Over the years, it has remained one of the most popular programming languages due to its unique features, which make it ideal for system programming, application development, and even embedded systems. In this article, we’ll explore the key features of C that make it an essential tool for programmers and why it continues to be a foundation for modern software development.
Key Features of C
C is distinguished by several features that set it apart from other programming languages. These features provide programmers with flexibility, control, and efficiency when developing software. Let’s take a closer look at the major features of C:
- Simplicity: C is a simple and straightforward language with a small number of keywords and a clean syntax. This makes it easy for beginners to learn while also allowing experienced programmers to write clear and efficient code. Despite its simplicity, C provides all the necessary tools for structured programming and system development.
- Portability: One of C's most valuable features is its portability. A program written in C can be run on different machines with little to no modification, making it a highly portable language across different hardware architectures and operating systems. This feature was key in the adoption of C for system programming, especially in UNIX and other operating systems.
- Efficiency: C is known for its ability to write highly efficient code. Since C provides direct access to memory and system resources, it enables developers to write programs that execute quickly and use minimal system resources. This makes C ideal for performance-critical applications such as operating systems, compilers, and embedded systems.
- Structured Programming: C supports structured programming techniques, which include the use of functions, loops, and conditionals to organize code into modular, reusable components. This makes programs easier to read, debug, and maintain, allowing for a more logical approach to problem-solving.
- Rich Library Support: C comes with a rich set of built-in libraries that provide standard functions for input/output, memory management, string manipulation, and mathematics. These libraries save time and effort by allowing developers to leverage pre-existing, tested code instead of writing everything from scratch.
- Low-Level Access: C allows programmers to access low-level memory through pointers, enabling them to directly manipulate data and hardware. This makes C an ideal language for writing system software, device drivers, and embedded applications where direct interaction with the hardware is required.
- Dynamic Memory Management: C provides the ability to dynamically allocate and free memory using functions like `malloc()`, `calloc()`, `realloc()`, and `free()`. This flexibility allows developers to manage memory usage efficiently, particularly in applications where the amount of memory needed changes during execution.
- Recursion: C supports recursive functions, which are functions that call themselves. Recursion is a powerful tool for solving problems that can be broken down into smaller, repetitive sub-problems, such as in sorting algorithms, tree traversal, and solving mathematical equations.
- Modularity: C programs can be divided into smaller, modular blocks using functions. Each function performs a specific task, allowing developers to break complex problems into manageable pieces. This modularity improves code readability, maintainability, and reusability.
- Extensibility: C is highly extensible, meaning that new features can be easily added to existing code. This makes it possible to build on top of C programs by adding new functionality without significantly altering the original codebase. Many modern programming languages, such as C++, Java, and C#, have been built by extending the core concepts of C.
Advantages of Using C
The features of C provide several advantages to programmers, making it an essential language for various applications. Some of the key advantages include:
- Speed and Performance: Programs written in C tend to be faster and more efficient due to the language's low-level capabilities and control over system resources. This makes it ideal for applications where performance is critical, such as operating systems, gaming, and embedded systems.
- Wide Adoption: Due to its portability and efficiency, C has been widely adopted across multiple industries, from telecommunications to aerospace. It remains one of the most widely used languages globally.
- Foundation for Learning Other Languages: Learning C provides a strong foundation for mastering other languages, particularly those that are influenced by C, such as C++, Java, and C#. Understanding C helps programmers easily transition to these languages due to the shared syntax and programming paradigms.
- Control Over System Resources: C offers fine-grained control over memory, CPU, and other system resources, making it suitable for system-level programming, where such control is necessary.
Conclusion
The C programming language continues to be a cornerstone of modern software development due to its simplicity, efficiency, and power. Its unique features, such as low-level access, portability, and modularity, make it a valuable tool for writing system software, embedded applications, and high-performance programs. Despite the rise of newer languages, C remains relevant, offering developers the flexibility and control needed to build efficient and reliable software. Mastering C is not only beneficial for system-level programming but also serves as a strong foundation for learning many of today’s most popular programming languages.
The Structure of a C Program: Building Blocks of C Programming
Understanding the structure of a C program is essential for writing clear, organized, and functional code. Every C program follows a specific structure, which helps in organizing the code efficiently and making it more readable. In this article, we’ll break down the typical structure of a C program, explain the key components, and demonstrate how they work together to form a functional program.
Basic Structure of a C Program
In C, a program is made up of various components that work together to perform a specific task. These components include headers, the main function, and statements or expressions. Below is the basic structure of a C program:
// Example of a basic C program structure
#include <stdio.h> // Preprocessor Directive
// Function Prototypes (if any)
int main() // Main Function
{
// Variable Declarations
int a, b, sum;
// Statements and Expressions
a = 5;
b = 10;
sum = a + b;
// Output
printf("Sum: %d\n", sum);
return 0; // Return statement
}
Let’s break down the key components of this structure:
1. Preprocessor Directives
The first part of a C program typically includes preprocessor directives. These are lines that begin with the #
symbol and are used to include files or define constants before the actual compilation of the code begins. The most common preprocessor directive is #include
, which is used to include standard or user-defined header files.
Example:
#include <stdio.h> // Includes the Standard Input/Output library
In this example, stdio.h
is the standard input/output library that contains functions like printf()
and scanf()
. Without this inclusion, the program wouldn't have access to these functions.
2. Function Declarations or Prototypes
If the program contains additional functions other than the main()
function, the function declarations or prototypes are placed after the preprocessor directives and before the main()
function. Function prototypes define the function’s return type, name, and parameters, allowing the compiler to understand how the function will be used in the program.
Example:
int add(int a, int b); // Function prototype for a function that adds two integers
3. The main()
Function
Every C program must have a main()
function because it is the starting point of the program’s execution. The main()
function can return an integer value (typically 0) to indicate the program's termination status. Inside the main()
function, the program’s logic is written in the form of statements, expressions, and function calls.
Example:
int main() // Main function definition
{
// Program logic goes here
return 0; // Indicates that the program ended successfully
}
4. Variable Declarations
Before using variables in C, they must be declared with a specific data type (e.g., int
, float
, char
). Variables store data that the program manipulates during its execution. Variable declarations are usually placed at the beginning of the main()
function or within other functions.
Example:
int a, b, sum; // Declaration of three integer variables
5. Statements and Expressions
The core logic of the program consists of statements and expressions that manipulate data, control the flow of execution, and perform calculations. Statements include assignments, function calls, control structures (such as loops and conditionals), and more.
Example:
a = 5; // Assigns the value 5 to the variable 'a'
b = 10; // Assigns the value 10 to the variable 'b'
sum = a + b; // Adds 'a' and 'b' and stores the result in 'sum'
6. Input/Output Operations
C programs often use input/output functions to interact with the user or display results. The most commonly used input/output functions are printf()
for displaying output and scanf()
for receiving input from the user. These functions are available in the stdio.h
library.
Example:
printf("Sum: %d\n", sum); // Prints the value of 'sum'
7. Return Statement
The return statement is used in the main()
function to return control back to the operating system. The most common return value is 0, which indicates successful completion. Non-zero values can be used to indicate errors or special conditions.
Example:
return 0; // Ends the program and returns 0 to indicate success
Conclusion
The structure of a C program is simple but powerful, consisting of preprocessor directives, the main()
function, variable declarations, statements, and optional functions. Understanding the layout of a C program is crucial for writing clean, organized, and functional code. By mastering the structure, programmers can focus on implementing the logic and functionality of their applications, making C one of the most efficient and widely used programming languages in the world.
Compiling and Executing C Programs: Turning Code into Action
Once you have written a C program, the next step is to compile and execute it. Compilation is the process of converting the human-readable source code into machine code that the computer's processor can execute. Understanding how to compile and execute C programs is essential for any C programmer. In this article, we will walk through the steps of compiling and executing a C program, explaining the process in detail and introducing you to the tools used in this process.
The Process of Compiling and Executing a C Program
In C programming, the process of turning code into an executable program involves several stages: preprocessing, compiling, assembling, and linking. Here’s an overview of each stage:
- Preprocessing: The preprocessor handles directives that begin with
#
, such as#include
and#define
. It replaces macros, includes header files, and prepares the code for compilation. - Compiling: The compiler translates the preprocessed code into assembly language. This step checks for syntax errors and converts high-level code into low-level instructions.
- Assembling: The assembler converts the assembly language code into machine code (binary), which the computer's processor can understand. This code is stored in an object file.
- Linking: The linker combines object files and libraries into a single executable file. It resolves references to external functions and variables, linking them to the appropriate memory addresses.
Once these stages are complete, the result is an executable file that can be run on the system. The entire process is handled by a compiler like GCC (GNU Compiler Collection) or any other C compiler.
Steps to Compile and Execute a C Program
To compile and execute a C program, you need to follow these steps:
Step 1: Writing the Code
The first step is writing your C code in a text editor. You can use any text editor, such as Notepad, Sublime Text, Visual Studio Code, or an integrated development environment (IDE) like Code::Blocks or Eclipse. Save the file with a .c
extension, such as program.c
.
// Example: A simple C program to add two numbers
#include <stdio.h>
int main()
{
int num1, num2, sum;
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("Sum: %d\n", sum);
return 0;
}
Step 2: Compiling the Code
Once your code is written and saved, you need to compile it using a compiler. The GCC compiler is widely used for compiling C programs. To compile a program using GCC, open your terminal or command prompt and navigate to the directory where your C file is saved. Use the following command:
gcc program.c -o program
Here’s what this command does:
- gcc: Invokes the GCC compiler.
- program.c: Specifies the source file to be compiled.
- -o program: Specifies the name of the output executable file. If you omit the
-o
option, the compiler will create an executable nameda.out
by default.
If the compilation is successful, the compiler will create an executable file named program
(or program.exe
on Windows). If there are any syntax errors in the code, the compiler will display error messages to help you identify and fix them.
Step 3: Executing the Program
After the program is successfully compiled, you can execute it. To run the program, use the following command in the terminal or command prompt:
./program
On Windows, the command would be:
program.exe
Once executed, the program will perform the task defined in the code. In our example, the program will prompt the user to enter two numbers and then display their sum.
Using an Integrated Development Environment (IDE)
While you can compile and execute C programs using the command line, many programmers prefer using an IDE. An IDE provides a more user-friendly interface that integrates code writing, compiling, and debugging into a single platform. Popular IDEs for C programming include Code::Blocks, Eclipse, and Visual Studio Code.
Here’s how to compile and run a program in Code::Blocks:
- Step 1: Open Code::Blocks and create a new project.
- Step 2: Write your C code in the editor.
- Step 3: Click the "Build" button to compile the code. Code::Blocks will automatically handle the compilation process for you.
- Step 4: Click the "Run" button to execute the compiled program.
Handling Compilation Errors
During the compilation process, you may encounter errors such as syntax errors, missing semicolons, undeclared variables, or type mismatches. The compiler will provide error messages that indicate the line number and nature of the error. Carefully read these messages, fix the issues, and recompile the program.
Conclusion
Compiling and executing a C program is a multi-step process that involves writing the code, using a compiler to translate the code into machine language, and then running the resulting executable. While the command-line approach is common, using an IDE can simplify the process by providing built-in tools for writing, compiling, and debugging your code. By understanding this process, you will gain the ability to turn your C programs into functioning software that interacts with users and performs useful tasks.
Variables and Data Types in C: Managing and Storing Data
In C programming, variables and data types are the core concepts that allow programmers to store and manipulate data effectively. Variables act as containers for data, while data types define the nature of the data that can be stored in those variables. Understanding how to declare variables and use the appropriate data types is essential for writing efficient and error-free programs. In this article, we will explore the basics of variables and data types in C, including their syntax, usage, and importance.
What is a Variable?
A variable is a named storage location in memory that holds a value which can be modified during the execution of a program. Variables allow us to store data, perform calculations, and manipulate values as needed. Each variable in C must be declared with a specific data type that determines the kind of data it can hold.
For example, if we want to store a whole number, we would declare a variable of type int
(integer). If we want to store a floating-point number, we would declare a variable of type float
. The variable's data type also determines the amount of memory allocated to it and the operations that can be performed on it.
Declaring and Initializing Variables
In C, variables must be declared before they are used. A variable declaration specifies the data type of the variable and its name. Optionally, variables can be initialized at the time of declaration by assigning them an initial value.
The general syntax for declaring a variable is:
data_type variable_name;
Here is an example of declaring and initializing variables:
int age; // Declaration of an integer variable
float height = 5.9; // Declaration and initialization of a float variable
char grade = 'A'; // Declaration and initialization of a char variable
In this example:
age
is an integer variable that will hold whole numbers.height
is a float variable that holds a floating-point number.grade
is a char variable that holds a single character.
Common Data Types in C
C offers several fundamental data types that can be used to store different kinds of data. These data types are divided into primary types, derived types, and user-defined types. Let’s explore the most commonly used primary data types:
- int: The
int
data type is used to store whole numbers (integers). The size of an integer can vary depending on the system, but it is typically 2 or 4 bytes. Example:
int number = 42;
float
data type is used to store single-precision floating-point numbers. It is typically 4 bytes in size and can store numbers with decimal points. Example:float temperature = 98.6;
double
data type is used to store double-precision floating-point numbers, providing greater precision than float
. It is typically 8 bytes in size. Example:double pi = 3.14159;
char
data type is used to store individual characters. It is typically 1 byte in size and can hold any single character, such as a letter, digit, or symbol. Example:char letter = 'A';
void
data type is used to indicate that a function does not return a value or that a pointer has no specific data type. Example:void myFunction(); // Function returns no value
Derived Data Types
In addition to primary data types, C provides derived data types, which are created using the primary data types. These include:
- Arrays: Collections of elements of the same data type stored in contiguous memory locations. Example:
int numbers[5]; // Array of 5 integers
int *ptr;
struct Person {
char name[50];
int age;
};
Modifiers in C
In C, data types can be modified using type modifiers such as short
, long
, signed
, and unsigned
. These modifiers alter the size and range of the data type.
- short: Reduces the size of an integer. Example:
short int smallNumber;
long int bigNumber;
unsigned int positiveNumber;
signed int number;
Memory Allocation for Data Types
The size of each data type in memory depends on the system architecture and the data type itself. Here is a typical allocation of memory for primary data types on a 32-bit system:
- int: 4 bytes
- float: 4 bytes
- double: 8 bytes
- char: 1 byte
Knowing the memory size of data types helps in optimizing programs, especially when working with large datasets or memory-constrained systems like embedded devices.
Conclusion
Variables and data types form the foundation of C programming, enabling the storage and manipulation of data in a structured and efficient way. By understanding how to declare variables, choose appropriate data types, and use type modifiers, programmers can write more efficient and optimized code. Mastering these concepts is essential for working effectively in C and other programming languages influenced by C.
Operators in C: Performing Operations on Data
Operators are special symbols in C that perform operations on variables and values. They are essential for writing expressions that manipulate data in C programs. Operators can be used to perform arithmetic, logic, comparison, assignment, and bitwise operations, among others. Understanding the different types of operators and how to use them is crucial for controlling the flow of a program and processing data effectively. In this article, we’ll explore the various operators available in C and their usage.
Types of Operators in C
C provides a wide range of operators, which can be categorized into the following types:
- Arithmetic Operators
- Relational (Comparison) Operators
- Logical Operators
- Assignment Operators
- Increment and Decrement Operators
- Bitwise Operators
- Conditional (Ternary) Operator
- Special Operators
1. Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations, such as addition, subtraction, multiplication, and division. These operators work on numerical data types, such as integers and floating-point numbers.
+
(Addition): Adds two operands. Example:
int sum = a + b;
-
(Subtraction): Subtracts the second operand from the first. Example:int difference = a - b;
*
(Multiplication): Multiplies two operands. Example:int product = a * b;
/
(Division): Divides the first operand by the second. Example:int quotient = a / b;
%
(Modulus): Returns the remainder when the first operand is divided by the second. Example:int remainder = a % b;
2. Relational (Comparison) Operators
Relational operators are used to compare two values or expressions. They return either true
(1) or false
(0) depending on the relationship between the operands.
==
(Equal to): Checks if two operands are equal. Example:
if (a == b)
!=
(Not equal to): Checks if two operands are not equal. Example:if (a != b)
>
(Greater than): Checks if the first operand is greater than the second. Example:if (a > b)
<
(Less than): Checks if the first operand is less than the second. Example:if (a < b)
>=
(Greater than or equal to): Checks if the first operand is greater than or equal to the second. Example:if (a >= b)
<=
(Less than or equal to): Checks if the first operand is less than or equal to the second. Example:if (a <= b)
3. Logical Operators
Logical operators are used to combine multiple conditions or expressions. They return true
or false
based on the logical relationship between the operands.
&&
(Logical AND): Returnstrue
if both operands are true. Example:
if (a > 0 && b > 0)
||
(Logical OR): Returns true
if at least one of the operands is true. Example:if (a > 0 || b > 0)
!
(Logical NOT): Reverses the logical state of the operand. Example:if (!a)
4. Assignment Operators
Assignment operators are used to assign values to variables. The basic assignment operator is =
, but there are also compound assignment operators that perform an operation and assign the result to the variable.
=
(Assignment): Assigns the value of the right operand to the left operand. Example:
a = 10;
+=
(Add and assign): Adds the right operand to the left operand and assigns the result to the left operand. Example:a += 5; // Equivalent to a = a + 5;
-=
(Subtract and assign): Subtracts the right operand from the left operand and assigns the result to the left operand. Example:a -= 3; // Equivalent to a = a - 3;
*=
(Multiply and assign): Multiplies the left operand by the right operand and assigns the result to the left operand. Example:a *= 2; // Equivalent to a = a * 2;
/=
(Divide and assign): Divides the left operand by the right operand and assigns the result to the left operand. Example:a /= 2; // Equivalent to a = a / 2;
%=
(Modulus and assign): Takes the modulus of the left operand by the right operand and assigns the result to the left operand. Example:a %= 3; // Equivalent to a = a % 3;
5. Increment and Decrement Operators
These operators are used to increase or decrease the value of a variable by 1. They are commonly used in loops and conditional statements.
++
(Increment): Increases the value of the operand by 1. Example:
a++;
--
(Decrement): Decreases the value of the operand by 1. Example:a--;
6. Bitwise Operators
Bitwise operators perform operations at the bit level. They are used to manipulate individual bits of integer data types.
&
(Bitwise AND): Performs a bitwise AND operation between two operands. Example:
a & b;
|
(Bitwise OR): Performs a bitwise OR operation between two operands. Example:a | b;
^
(Bitwise XOR): Performs a bitwise XOR operation between two operands. Example:a ^ b;
~
(Bitwise NOT): Inverts the bits of the operand. Example:~a;
<<
(Left shift): Shifts the bits of the first operand left by the number of positions specified by the second operand. Example:a << 2;
>>
(Right shift): Shifts the bits of the first operand right by the number of positions specified by the second operand. Example:a >> 2;
7. Conditional (Ternary) Operator
The conditional operator is a shorthand way of writing an if-else
statement. It is represented by the symbols ?
and :
.
condition ? expression1 : expression2;
If the condition is true, the value of expression1
is returned; otherwise, the value of expression2
is returned. Example:
int max = (a > b) ? a : b;
8. Special Operators
C also includes special operators such as the comma operator, sizeof operator, and pointer operators.
,
(Comma Operator): Used to separate multiple expressions in a statement. Example:
int a = 1, b = 2, c = 3;
sizeof
(Sizeof Operator): Returns the size of a data type or variable in bytes. Example:sizeof(int);
&
(Address Operator): Returns the memory address of a variable. Example:&a;
*
(Pointer Dereference Operator): Used to access the value stored at the address a pointer points to. Example:*ptr;
Conclusion
Operators in C allow programmers to perform a wide range of operations on data, from basic arithmetic to complex bitwise manipulations. Mastering the various operators in C is essential for writing efficient and powerful code. Whether you are performing calculations, making decisions, or manipulating bits, understanding how to use operators effectively will enable you to solve a variety of programming problems.
Input and Output Functions in C: Interacting with Users and Displaying Results
Input and output (I/O) functions in C are essential for interacting with the user and displaying the results of a program's execution. The standard input and output library in C, <stdio.h>
, provides a range of functions for receiving input from the user and outputting data to the console. Understanding how to use these functions effectively is crucial for writing interactive programs. In this article, we will explore the commonly used input and output functions in C and how they work.
Common Input Functions
C provides several functions for receiving input from the user, with scanf()
and getchar()
being the most commonly used. These functions allow the program to read data from standard input (usually the keyboard).
1. scanf()
The scanf()
function is used to read formatted input from the user. It allows the user to enter data that can be stored in variables. The syntax of scanf()
is as follows:
scanf("format_specifiers", &variable1, &variable2, ...);
The format specifiers determine the type of data to be read (e.g., %d
for integers, %f
for floats, %c
for characters, etc.). The addresses of the variables where the input will be stored are passed using the address-of operator (&
).
Example:
int age;
float height;
printf("Enter your age and height: ");
scanf("%d %f", &age, &height);
printf("You entered: Age = %d, Height = %.2f\n", age, height);
In this example, the user is prompted to enter their age and height, which are then stored in the respective variables and displayed back to the user.
2. getchar()
The getchar()
function reads a single character from the standard input (keyboard). It does not require format specifiers and returns the character entered by the user. The syntax is as follows:
char c = getchar();
Example:
char letter;
printf("Enter a character: ");
letter = getchar();
printf("You entered: %c\n", letter);
This example demonstrates how to use getchar()
to read a single character from the user and display it on the screen.
3. gets()
(Deprecated)
The gets()
function is used to read a string (sequence of characters) from the user until a newline character is encountered. However, gets()
has been deprecated due to security issues, such as buffer overflows. It is recommended to use fgets()
instead for reading strings safely.
4. fgets()
The fgets()
function reads a string from the input, including spaces, until a newline character or the end of the buffer is reached. The syntax is as follows:
fgets(string, size, stdin);
Example:
char name[50];
printf("Enter your name: ");
fgets(name, sizeof(name), stdin);
printf("Your name is: %s", name);
In this example, fgets()
safely reads a string from the user and stores it in the name
variable.
Common Output Functions
C provides several functions for sending output to the console. The most commonly used output functions are printf()
and putchar()
. These functions are used to display text, variables, and the results of expressions.
1. printf()
The printf()
function is used to print formatted output to the console. It allows you to display text, variables, and the results of expressions. The syntax of printf()
is as follows:
printf("format_string", variable1, variable2, ...);
The format string contains placeholders (format specifiers) that indicate the type of data to be printed (e.g., %d
for integers, %f
for floats, %c
for characters, %s
for strings, etc.). The variables to be printed are listed after the format string.
Example:
int num = 10;
float price = 99.99;
printf("Number: %d, Price: %.2f\n", num, price);
In this example, printf()
is used to print an integer and a floating-point number in a formatted manner.
2. putchar()
The putchar()
function is used to print a single character to the console. The syntax is as follows:
putchar(character);
Example:
char letter = 'A';
putchar(letter);
putchar('\n'); // Prints a newline character
This example demonstrates how to use putchar()
to print a single character to the console.
3. puts()
The puts()
function is used to print a string followed by a newline character. It is similar to printf()
but is used specifically for strings and does not require format specifiers.
puts(string);
Example:
char message[] = "Hello, World!";
puts(message);
In this example, puts()
prints the string stored in the message
array, followed by a newline.
Formatted Output with printf()
The printf()
function is highly flexible due to its use of format specifiers, which allow you to control how data is displayed. Here are some common format specifiers:
%d
or%i
for integers%f
for floating-point numbers%c
for characters%s
for strings%.nf
to control the number of decimal places (e.g.,%.2f
)
Example:
int num = 25;
float pi = 3.14159;
printf("Integer: %d, Float: %.2f\n", num, pi);
Conclusion
Input and output functions in C allow you to interact with users and display results. The scanf()
and printf()
functions are the most commonly used for receiving input and producing output, respectively. Mastering these functions is essential for writing interactive and user-friendly C programs that take input from the user and provide meaningful output. Understanding how to format input and output properly ensures that your program communicates effectively with users.
Control Structures in C: if, else, switch, and Loops
Control structures in C allow programmers to control the flow of execution based on certain conditions or to repeat sections of code. These structures are essential for implementing logic and decision-making in programs. The most commonly used control structures in C include conditional statements (if
, else
, switch
) and loops (for
, while
, do-while
). In this article, we’ll explore these control structures and how to use them effectively.
Conditional Statements
Conditional statements are used to perform different actions based on certain conditions. C provides three main types of conditional statements: if
, else
, and switch
.
1. The if
Statement
The if
statement allows the program to execute a block of code only if a specified condition is true. The syntax of the if
statement is as follows:
if (condition) {
// Code to execute if the condition is true
}
Example:
int num = 10;
if (num > 0) {
printf("The number is positive.\n");
}
In this example, the code inside the if
block will only execute if the condition num > 0
is true.
2. The else
Statement
The else
statement is used to define an alternative block of code that will execute if the condition in the if
statement is false. The syntax of the else
statement is as follows:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Example:
int num = -5;
if (num > 0) {
printf("The number is positive.\n");
} else {
printf("The number is non-positive.\n");
}
In this example, if the number is not greater than zero, the code inside the else
block will be executed.
3. The else if
Ladder
The else if
ladder allows you to check multiple conditions sequentially. Once a condition is true, the corresponding block of code will execute, and the rest of the ladder will be skipped.
if (condition1) {
// Code for condition1
} else if (condition2) {
// Code for condition2
} else {
// Code if none of the above conditions are true
}
Example:
int num = 0;
if (num > 0) {
printf("The number is positive.\n");
} else if (num == 0) {
printf("The number is zero.\n");
} else {
printf("The number is negative.\n");
}
In this example, the program checks multiple conditions and executes the appropriate block of code based on the value of num
.
4. The switch
Statement
The switch
statement is used to execute one block of code among many based on the value of a variable or expression. It is an alternative to the else if
ladder when dealing with multiple possible values for a single variable. The syntax of the switch
statement is as follows:
switch (expression) {
case value1:
// Code for value1
break;
case value2:
// Code for value2
break;
// More cases...
default:
// Code if no case matches
}
Example:
int day = 2;
switch (day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
default:
printf("Invalid day\n");
}
In this example, the program will print "Tuesday" because the value of day
is 2.
Loops
Loops are control structures that allow the program to execute a block of code repeatedly, either for a specific number of iterations or until a condition is met. C provides three types of loops: for
, while
, and do-while
.
1. The for
Loop
The for
loop is used to repeat a block of code for a specified number of times. The syntax of the for
loop is as follows:
for (initialization; condition; increment) {
// Code to execute
}
Example:
for (int i = 0; i < 5; i++) {
printf("Iteration %d\n", i);
}
In this example, the loop will execute five times, printing the iteration number each time.
2. The while
Loop
The while
loop is used to repeat a block of code as long as a specified condition is true. The syntax of the while
loop is as follows:
while (condition) {
// Code to execute
}
Example:
int i = 0;
while (i < 5) {
printf("Iteration %d\n", i);
i++;
}
In this example, the loop will execute as long as i
is less than 5.
3. The do-while
Loop
The do-while
loop is similar to the while
loop, but it guarantees that the block of code will be executed at least once, even if the condition is false. The syntax of the do-while
loop is as follows:
do {
// Code to execute
} while (condition);
Example:
int i = 0;
do {
printf("Iteration %d\n", i);
i++;
} while (i < 5);
In this example, the loop will execute five times, just like the previous loops. However, the condition is checked after the code is executed.
Breaking and Continuing Loops
C also provides break
and continue
statements to control the flow of loops.
break
: Thebreak
statement is used to exit the loop immediately, regardless of the loop's condition. It is commonly used inswitch
statements and loops.continue
: Thecontinue
statement skips the remaining code inside the loop for the current iteration and moves to the next iteration.
Conclusion
Control structures in C, including if
, else
, switch
, and loops, allow you to control the flow of your program and make it more dynamic and responsive. By using these control structures effectively, you can implement decision-making, repetition, and branching logic in your C programs, enabling you to write more flexible and efficient code.
Functions in C: Reusable Blocks of Code
In C programming, functions are reusable blocks of code designed to perform a specific task. Functions help make code more modular, organized, and easier to maintain. By breaking a large program into smaller, manageable parts, functions enable you to reuse code, improve readability, and reduce redundancy. In this article, we will explore the concept of functions in C, including how to declare, define, and call functions.
What is a Function?
A function is a self-contained block of code that performs a specific task. Once defined, a function can be called multiple times from different parts of the program, enabling code reuse and reducing redundancy. Functions can take input through parameters, perform computations or operations, and optionally return a result.
There are two main types of functions in C:
- Standard Library Functions: These are built-in functions provided by C's standard libraries, such as
printf()
andscanf()
. You can use these functions directly without needing to define them. - User-Defined Functions: These are functions that you define yourself to perform specific tasks based on the requirements of your program.
Function Declaration (Prototype)
Before you define a function, you need to declare it (also called providing a function prototype) so that the compiler knows about the function's name, return type, and parameters before it is used. The syntax for declaring a function is as follows:
return_type function_name(parameter_list);
Example:
int add(int, int);
In this example, int
is the return type of the function, add
is the function name, and (int, int)
specifies that the function takes two integer parameters.
Function Definition
The function definition contains the actual code that performs the task when the function is called. The syntax for defining a function is as follows:
return_type function_name(parameter_list) {
// Function body: statements to execute
return value; // Optional: return statement
}
Example:
int add(int a, int b) {
int sum = a + b;
return sum;
}
In this example, the add
function takes two integers as input, adds them, and returns the result.
Calling a Function
Once a function is declared and defined, it can be called (invoked) in your main program or in other functions. When a function is called, the program's execution jumps to the function's body, executes its code, and then returns to the point where it was called.
The syntax for calling a function is as follows:
function_name(arguments);
Example:
int result = add(5, 3); // Calling the add function
printf("The sum is: %d\n", result);
In this example, the add
function is called with the arguments 5
and 3
, and the result is stored in the variable result
, which is then printed to the console.
Return Type of Functions
The return type of a function specifies the type of value that the function will return to the caller. If a function does not return any value, its return type should be void
. The return type must be compatible with the value that is returned using the return
statement.
Example:
void displayMessage() {
printf("Hello, World!\n");
}
int getMax(int a, int b) {
return (a > b) ? a : b;
}
In this example, displayMessage()
has a void
return type and does not return any value, while getMax()
returns the larger of two integers.
Passing Arguments to Functions
Functions can accept input values through parameters, which are variables defined in the function declaration and definition. These parameters receive the values passed to the function when it is called. There are two common ways to pass arguments to functions in C:
- Pass by Value: When arguments are passed by value, the function receives a copy of the actual data. Any changes made to the parameters inside the function do not affect the original data.
- Pass by Reference: When arguments are passed by reference (using pointers), the function receives the memory address of the data, and changes made to the parameters inside the function affect the original data.
Example of Pass by Value:
void modifyValue(int x) {
x = x + 10;
printf("Inside function: %d\n", x);
}
int main() {
int num = 5;
modifyValue(num);
printf("Outside function: %d\n", num);
return 0;
}
In this example, the value of num
remains unchanged outside the function because the function only modifies a copy of the original value.
Recursive Functions
A recursive function is a function that calls itself to solve smaller instances of a problem. Recursion is a powerful tool for solving problems that can be divided into subproblems of the same type, such as calculating factorials or performing tree traversals.
Example of a Recursive Function:
int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
int main() {
int num = 5;
printf("Factorial of %d is %d\n", num, factorial(num));
return 0;
}
In this example, the factorial
function calls itself recursively to calculate the factorial of a number.
Conclusion
Functions in C are essential for breaking down programs into smaller, reusable blocks of code. By using functions, you can write cleaner, more maintainable, and more efficient programs. Whether you are using standard library functions or defining your own, understanding how to declare, define, and call functions is crucial for mastering C programming.
Arrays and Strings in C: Managing Collections of Data
Arrays and strings are essential data structures in C that allow you to manage collections of data efficiently. An array is a collection of elements of the same type stored in contiguous memory locations, while a string is a special type of array used to store sequences of characters. Both arrays and strings enable you to store and manipulate data effectively in C programs. In this article, we will explore the concept of arrays and strings, how to declare, initialize, and use them in C.
What is an Array?
An array is a collection of elements of the same data type, such as integers, floats, or characters, stored in contiguous memory locations. Arrays allow you to store multiple values in a single variable and access them using an index. The size of an array is fixed at the time of its declaration, and each element is identified by an index starting from 0.
The syntax for declaring an array in C is as follows:
data_type array_name[size];
Example:
int numbers[5]; // Declares an array of 5 integers
Initializing Arrays
You can initialize arrays at the time of declaration by providing a comma-separated list of values enclosed in curly braces. The size of the array can be omitted if it is determined by the number of elements in the initializer list.
Example:
int numbers[5] = {1, 2, 3, 4, 5}; // Array initialized with 5 elements
float temperatures[] = {98.6, 101.2, 99.4}; // Size is determined by the number of elements
In this example, the array numbers
is initialized with five integers, and the array temperatures
is initialized with three floating-point values.
Accessing Array Elements
Array elements are accessed using their index, which starts at 0. To access or modify a specific element, use the array name followed by the index in square brackets.
Example:
int numbers[5] = {1, 2, 3, 4, 5};
printf("%d\n", numbers[0]); // Prints the first element (1)
numbers[2] = 10; // Modifies the third element (changes 3 to 10)
printf("%d\n", numbers[2]); // Prints the modified element (10)
In this example, the first element of the numbers
array is printed, and the third element is modified.
Multidimensional Arrays
C also supports multidimensional arrays, such as 2D arrays, which are arrays of arrays. A common use of 2D arrays is to represent matrices or grids.
The syntax for declaring a 2D array is as follows:
data_type array_name[rows][columns];
Example:
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
printf("%d\n", matrix[1][2]); // Prints the element in the second row and third column (6)
In this example, a 3x3 matrix is initialized, and the element in the second row and third column is printed.
What is a String?
A string in C is a sequence of characters terminated by a null character ('\0'
). Strings are stored in arrays of characters, where the null character signifies the end of the string. Unlike arrays of other data types, strings require a special handling due to the presence of the null character.
The syntax for declaring a string is as follows:
char string_name[size];
Example:
char name[10] = "John"; // Declares a string with a size of 10 and initializes it with "John"
In this example, the string name
is initialized with the characters 'J', 'o', 'h', 'n', followed by the null character '\0'
to mark the end of the string.
Initializing Strings
You can initialize strings using character arrays or string literals. String literals automatically include the null character at the end.
Example:
char greeting[] = "Hello, World!"; // The null character is automatically added
char word[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; // Explicitly adding the null character
In this example, the string greeting
is initialized using a string literal, while the string word
is initialized using individual characters with an explicit null character.
Common String Functions
C provides several standard library functions to manipulate strings. These functions are available in the <string.h>
header file.
strlen()
: Returns the length of a string (excluding the null character).strcpy()
: Copies one string into another.strcmp()
: Compares two strings lexicographically.strcat()
: Concatenates two strings.
Example:
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello";
char str2[] = "World";
// Concatenate str2 to str1
strcat(str1, str2);
printf("Concatenated String: %s\n", str1); // Output: HelloWorld
// Calculate the length of str1
printf("Length of str1: %lu\n", strlen(str1)); // Output: 10
return 0;
}
In this example, the strcat()
function concatenates str2
to str1
, and strlen()
calculates the length of the concatenated string.
Passing Arrays and Strings to Functions
When passing arrays and strings to functions, you pass the base address (pointer) of the array or string. This allows the function to access and modify the elements of the array or string.
Example of Passing an Array to a Function:
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
int numbers[] = {1, 2, 3, 4, 5};
int size = sizeof(numbers) / sizeof(numbers[0]);
printArray(numbers, size); // Output: 1 2 3 4 5
return 0;
}
In this example, the array numbers
is passed to the printArray()
function, which prints all its elements.
Conclusion
Arrays and strings in C are powerful tools for managing collections of data. Arrays allow you to store multiple values of the same type, while strings enable the manipulation of sequences of characters. Understanding how to declare, initialize, and manipulate arrays and strings is essential for solving complex programming problems efficiently in C.
Pointers in C: Memory Management and Address Manipulation
Pointers are a powerful feature of C programming that allow you to directly manage and manipulate memory. A pointer is a variable that stores the memory address of another variable. Using pointers, you can access and modify the values stored at specific memory locations, pass large data structures to functions efficiently, and implement dynamic memory allocation. In this article, we will explore the concept of pointers in C, including how to declare, initialize, and use them effectively.
What is a Pointer?
A pointer is a special variable that holds the memory address of another variable. Instead of storing a data value directly, a pointer stores the location (address) in memory where the data is stored. This allows for efficient memory access and manipulation, especially when working with large data structures such as arrays and strings.
The syntax for declaring a pointer is as follows:
data_type *pointer_name;
Example:
int *ptr; // Declares a pointer to an integer
In this example, ptr
is a pointer that can store the memory address of an integer variable.
Initializing Pointers
Pointers are initialized by assigning them the address of a variable. The address-of operator &
is used to obtain the address of a variable, which is then stored in the pointer.
Example:
int num = 10;
int *ptr = # // Pointer initialized with the address of num
In this example, the pointer ptr
is initialized with the address of the integer variable num
. Now, ptr
holds the memory address of num
.
Dereferencing Pointers
Dereferencing a pointer means accessing the value stored at the memory location pointed to by the pointer. This is done using the dereference operator *
.
Example:
int num = 10;
int *ptr = #
printf("Value of num: %d\n", *ptr); // Dereferencing the pointer to access the value of num
In this example, *ptr
accesses the value stored at the memory location pointed to by ptr
, which is the value of num
.
Pointer Arithmetic
Pointers in C can be incremented, decremented, and manipulated using arithmetic operations. Pointer arithmetic is based on the size of the data type to which the pointer points. When a pointer is incremented, it moves to the next memory location for that data type.
Example:
int arr[] = {10, 20, 30, 40, 50};
int *ptr = arr; // Points to the first element of the array
printf("Value at ptr: %d\n", *ptr); // Output: 10
ptr++;
printf("Value at ptr: %d\n", *ptr); // Output: 20
In this example, the pointer ptr
initially points to the first element of the array arr
. After incrementing the pointer, it points to the second element.
Pointers and Arrays
Arrays and pointers are closely related in C. The name of an array acts as a pointer to its first element, so you can use pointers to traverse arrays. This makes pointer manipulation especially useful when working with arrays.
Example:
int arr[] = {1, 2, 3, 4, 5};
int *ptr = arr; // Points to the first element of the array
for (int i = 0; i < 5; i++) {
printf("Value at arr[%d] = %d\n", i, *(ptr + i));
}
In this example, the pointer ptr
is used to traverse the array arr
by incrementing the pointer and dereferencing it to access the array elements.
Pointers to Pointers
In C, you can have pointers that point to other pointers. This is known as a pointer to a pointer (or double pointer). Pointers to pointers are often used in dynamic memory allocation and multi-level data structures.
Example:
int num = 10;
int *ptr = #
int **ptr2 = &ptr; // Pointer to pointer
printf("Value of num: %d\n", **ptr2); // Dereferencing twice to get the value of num
In this example, ptr2
is a pointer to ptr
, and **ptr2
accesses the value of num
.
Dynamic Memory Allocation
Pointers are essential for dynamic memory allocation, where memory is allocated at runtime. The functions malloc()
, calloc()
, realloc()
, and free()
from the <stdlib.h>
library are used for allocating and deallocating memory dynamically.
Using malloc()
The malloc()
function allocates a specified number of bytes and returns a pointer to the allocated memory. If the memory allocation fails, it returns NULL
.
Example:
int *ptr;
ptr = (int*)malloc(5 * sizeof(int)); // Allocates memory for 5 integers
if (ptr == NULL) {
printf("Memory not allocated.\n");
} else {
for (int i = 0; i < 5; i++) {
ptr[i] = i + 1;
}
for (int i = 0; i < 5; i++) {
printf("%d ", ptr[i]);
}
free(ptr); // Deallocates the memory
}
In this example, memory is dynamically allocated for five integers, initialized with values, and then deallocated using the free()
function.
Pointer Function Parameters
Pointers allow you to pass large data structures such as arrays, strings, and structs to functions efficiently. When a pointer is passed to a function, the function can modify the original data because it works with the memory address rather than a copy of the data.
Example of Passing a Pointer to a Function:
void increment(int *num) {
(*num)++; // Increment the value pointed to by num
}
int main() {
int value = 10;
increment(&value); // Passing the address of value
printf("Updated value: %d\n", value); // Output: 11
return 0;
}
In this example, the pointer num
is passed to the function increment()
, allowing the function to modify the original variable value
.
Advantages of Pointers
- Efficient Memory Access: Pointers allow you to access and manipulate memory locations directly.
- Dynamic Memory Allocation: Pointers are essential for dynamic memory management, enabling the creation of data structures like linked lists and trees.
- Efficient Parameter Passing: Pointers allow functions to modify data directly without creating copies, reducing memory overhead.
- Flexibility: Pointers enable complex data structures and algorithms, such as dynamic arrays and recursive functions.
Disadvantages of Pointers
- Complexity: Pointers can be difficult to understand and manage, leading to bugs such as dangling pointers and memory leaks.
- Memory Management: Improper use of pointers can result in memory leaks, segmentation faults, and other runtime errors.
- Security Risks: Pointers can be misused to access and modify arbitrary memory locations, leading to security vulnerabilities.
Conclusion
Pointers are a crucial part of C programming, providing direct access to memory and enabling efficient manipulation of data structures. Understanding how to declare, initialize, and use pointers effectively is essential for writing powerful and efficient C programs. However, careful management is required to avoid common pitfalls such as memory leaks and pointer-related errors.
Structures and Unions in C: Grouping Data Efficiently
Structures and unions are user-defined data types in C that allow you to group different types of data under a single name. They are used to represent complex data models, such as records in a database or elements in a linked list. While both structures and unions group data, they differ in how they store and manage memory. In this article, we will explore the concepts of structures and unions in C, including how to declare, define, and use them effectively.
What is a Structure?
A structure in C is a user-defined data type that allows you to group different types of variables under a single name. Each variable within the structure is called a member, and they can be of different data types. Structures are commonly used to represent records, such as a student's information, an employee's data, or a geometric point.
The syntax for defining a structure is as follows:
struct structure_name {
data_type member1;
data_type member2;
// more members...
};
Example:
struct Student {
char name[50];
int roll_number;
float marks;
};
In this example, the structure Student
has three members: name
(an array of characters), roll_number
(an integer), and marks
(a floating-point number).
Declaring and Initializing Structures
After defining a structure, you can declare variables of that structure type and initialize them. Structure variables can be initialized during declaration or by assigning values to the members individually.
Example:
struct Student student1 = {"John Doe", 101, 95.5}; // Initialize during declaration
struct Student student2; // Declare structure variable
student2.roll_number = 102; // Assign values to members
strcpy(student2.name, "Jane Smith");
student2.marks = 89.0;
In this example, the structure variable student1
is initialized at the time of declaration, while the members of student2
are assigned values individually.
Accessing Structure Members
You can access and modify the members of a structure using the dot operator (.
). The structure variable is followed by the dot operator and the member name.
Example:
printf("Name: %s\n", student1.name);
printf("Roll Number: %d\n", student1.roll_number);
printf("Marks: %.2f\n", student1.marks);
student1.marks = 97.0; // Modifying the marks of student1
In this example, the members of the structure student1
are accessed and printed, and the marks
member is updated.
Structures as Function Parameters
Structures can be passed to functions either by value (passing a copy of the structure) or by reference (passing a pointer to the structure). Passing by reference is more efficient when dealing with large structures.
Example of Passing Structure by Value:
void printStudent(struct Student s) {
printf("Name: %s\n", s.name);
printf("Roll Number: %d\n", s.roll_number);
printf("Marks: %.2f\n", s.marks);
}
int main() {
struct Student student1 = {"John Doe", 101, 95.5};
printStudent(student1); // Passing structure by value
return 0;
}
In this example, the structure student1
is passed to the printStudent
function by value, meaning a copy of the structure is passed to the function.
Pointers to Structures
You can use pointers to structures to access and manipulate structure members. This is particularly useful when passing structures to functions by reference.
Example:
void updateMarks(struct Student *s, float new_marks) {
s->marks = new_marks; // Using the arrow operator to access members
}
int main() {
struct Student student1 = {"John Doe", 101, 95.5};
updateMarks(&student1, 98.5); // Passing a pointer to the structure
printf("Updated Marks: %.2f\n", student1.marks);
return 0;
}
In this example, the structure pointer s
is used to update the marks of student1
using the arrow operator (->
).
What is a Union?
A union is similar to a structure in that it can store multiple members of different data types. However, in a union, all members share the same memory location, meaning that only one member can hold a value at any given time. Unions are useful when you need to store one of many possible data types, but not simultaneously.
The syntax for defining a union is as follows:
union union_name {
data_type member1;
data_type member2;
// more members...
};
Example:
union Data {
int i;
float f;
char str[20];
};
In this example, the union Data
can store either an integer, a floating-point number, or a string, but only one at a time, since all members share the same memory space.
Initializing and Accessing Union Members
Just like structures, unions can be initialized during declaration or by assigning values to members individually. However, since all members share the same memory, assigning a value to one member will overwrite any previous value.
Example:
union Data data;
data.i = 10; // Assign value to integer member
printf("Integer: %d\n", data.i);
data.f = 220.5; // Assign value to float member (overwrites integer)
printf("Float: %.2f\n", data.f);
strcpy(data.str, "Hello, World!"); // Assign value to string member (overwrites float)
printf("String: %s\n", data.str);
In this example, the union data
is used to store an integer, a float, and a string. However, only one value is stored at a time, and each new assignment overwrites the previous value.
Differences Between Structures and Unions
- Memory Allocation: In structures, each member has its own memory space, while in unions, all members share the same memory space.
- Usage: Structures are used when you need to store multiple values simultaneously, while unions are used when you need to store one of many possible values at different times.
Conclusion
Structures and unions in C provide powerful ways to group and manage different types of data. Structures allow you to store multiple values of different types together, making them ideal for modeling complex data structures. Unions, on the other hand, enable efficient use of memory when you need to store one of several possible values at different times. Understanding when and how to use structures and unions effectively is essential for managing data in C programs.
Dynamic Memory Allocation in C: Managing Memory Efficiently
Dynamic memory allocation in C allows programs to request memory at runtime, making it possible to handle data whose size is not known at compile time. This feature enables efficient memory management, especially when working with data structures like linked lists, trees, and arrays whose sizes may change during execution. Dynamic memory allocation functions are part of the <stdlib.h>
library, and they allow you to allocate and deallocate memory as needed. In this article, we will explore the concept of dynamic memory allocation in C, the functions used for memory management, and best practices for using them.
Why Use Dynamic Memory Allocation?
In statically allocated memory, the size of data structures like arrays must be known at compile time. This can be limiting when the size of the data is unpredictable. Dynamic memory allocation solves this problem by allowing memory to be allocated on the fly, at runtime, enabling you to manage memory efficiently based on the program’s needs.
Dynamic memory allocation is essential in several scenarios, such as:
- When you need to allocate memory for data structures whose size is unknown at compile time.
- When the memory requirements may change during the program’s execution (e.g., linked lists, dynamic arrays).
- When efficient memory usage is required for large datasets.
Dynamic Memory Allocation Functions
C provides several functions for dynamic memory allocation, which are declared in the <stdlib.h>
library:
malloc()
: Allocates a specified number of bytes and returns a pointer to the allocated memory.calloc()
: Allocates memory for an array of elements, initializes the allocated memory to zero, and returns a pointer to the memory.realloc()
: Reallocates memory, changing the size of previously allocated memory blocks.free()
: Deallocates memory that was previously allocated, freeing up resources.
Using malloc()
The malloc()
function is used to allocate a specified number of bytes in memory. It returns a pointer to the allocated memory, which can be cast to the appropriate data type. If the allocation fails, malloc()
returns NULL
.
Syntax:
void* malloc(size_t size);
Example:
int *ptr = (int*)malloc(5 * sizeof(int)); // Allocate memory for 5 integers
if (ptr == NULL) {
printf("Memory allocation failed\n");
} else {
for (int i = 0; i < 5; i++) {
ptr[i] = i + 1;
printf("%d ", ptr[i]);
}
free(ptr); // Deallocate memory
}
In this example, memory is allocated dynamically for five integers using malloc()
. If the allocation is successful, the memory is used, and then it is freed using free()
.
Using calloc()
The calloc()
function allocates memory for an array of elements and initializes all bytes to zero. This function is useful when you need zero-initialized memory. The function returns a pointer to the allocated memory or NULL
if the allocation fails.
Syntax:
void* calloc(size_t num, size_t size);
Example:
int *ptr = (int*)calloc(5, sizeof(int)); // Allocate memory for 5 integers, initialized to 0
if (ptr == NULL) {
printf("Memory allocation failed\n");
} else {
for (int i = 0; i < 5; i++) {
printf("%d ", ptr[i]); // All values will be 0
}
free(ptr); // Deallocate memory
}
In this example, memory is allocated for five integers using calloc()
, and each element is automatically initialized to zero.
Using realloc()
The realloc()
function is used to resize a previously allocated memory block. It either expands or shrinks the memory allocation, depending on the new size requested. If the memory block cannot be resized in place, a new block is allocated, and the existing data is copied to it.
Syntax:
void* realloc(void* ptr, size_t new_size);
Example:
int *ptr = (int*)malloc(3 * sizeof(int)); // Initial allocation for 3 integers
if (ptr == NULL) {
printf("Memory allocation failed\n");
} else {
for (int i = 0; i < 3; i++) {
ptr[i] = i + 1;
}
ptr = (int*)realloc(ptr, 5 * sizeof(int)); // Resize to hold 5 integers
if (ptr == NULL) {
printf("Memory reallocation failed\n");
} else {
for (int i = 3; i < 5; i++) {
ptr[i] = i + 1;
}
for (int i = 0; i < 5; i++) {
printf("%d ", ptr[i]); // Output: 1 2 3 4 5
}
}
free(ptr); // Deallocate memory
}
In this example, memory is initially allocated for three integers, and then resized using realloc()
to hold five integers. The values are updated, and the resized array is printed.
Deallocating Memory with free()
Once dynamically allocated memory is no longer needed, it must be deallocated using the free()
function. Failing to free dynamically allocated memory results in memory leaks, where memory remains occupied and unavailable for future use.
Syntax:
void free(void* ptr);
Example:
int *ptr = (int*)malloc(5 * sizeof(int));
if (ptr != NULL) {
// Use the memory
free(ptr); // Deallocate memory when done
}
In this example, the dynamically allocated memory is deallocated using free()
, making it available for future use.
Best Practices for Dynamic Memory Allocation
- Check for
NULL
: Always check the return value of memory allocation functions to ensure the memory was successfully allocated. If the return value isNULL
, handle the error appropriately. - Use
free()
: Always free dynamically allocated memory when it is no longer needed to avoid memory leaks. - Use
realloc()
with Caution: When usingrealloc()
, check if the returned pointer isNULL
. If the reallocation fails, the original memory block remains unaffected. - Initialize Pointers: Always initialize pointers to
NULL
when declaring them, and only use them after ensuring they point to valid memory.
Conclusion
Dynamic memory allocation in C provides flexibility in managing memory during runtime, enabling the creation of dynamic data structures such as linked lists, trees, and dynamic arrays. By using functions like malloc()
, calloc()
, realloc()
, and free()
, you can allocate and deallocate memory as needed, making your programs more efficient and adaptable. Proper management of dynamically allocated memory is crucial to avoiding memory leaks and ensuring optimal performance in C applications.
File Handling in C: Working with Files
File handling in C allows programs to store and retrieve data from files, making it possible to save data persistently beyond the runtime of the program. Whether reading from or writing to files, file handling is an essential feature in C programming that enables data management across sessions. In this article, we will explore how to open, read, write, and close files in C, using the functions provided in the C Standard Library.
Why File Handling?
File handling is crucial for applications that need to persist data, such as databases, logs, configuration files, and more. Without file handling, data would be lost when the program terminates. By working with files, a program can store data, retrieve it later, and share it across multiple runs of the program.
In C, file handling is achieved through a set of functions provided in the <stdio.h>
library. These functions enable you to perform various operations such as reading from files, writing to files, and closing files after use.
File Operations in C
The basic file operations in C include:
fopen()
: Opens a file for reading or writing.fclose()
: Closes an open file.fprintf()
: Writes formatted data to a file.fscanf()
: Reads formatted data from a file.fgetc()
: Reads a character from a file.fputc()
: Writes a character to a file.fgets()
: Reads a string from a file.fputs()
: Writes a string to a file.
Opening a File: fopen()
The fopen()
function is used to open a file for reading or writing. It returns a pointer of type FILE*
that is used to access the file. If the file cannot be opened, fopen()
returns NULL
. Files can be opened in different modes, such as reading, writing, or appending.
Syntax:
FILE* fopen(const char* filename, const char* mode);
The mode
parameter specifies how the file should be opened:
"r"
: Open a file for reading (the file must exist)."w"
: Open a file for writing (creates a new file or truncates the existing file)."a"
: Open a file for appending (creates a new file if it doesn’t exist)."r+"
: Open a file for both reading and writing (the file must exist)."w+"
: Open a file for both reading and writing (creates a new file or truncates the existing file)."a+"
: Open a file for both reading and appending (creates a new file if it doesn’t exist).
Example:
FILE* file_ptr = fopen("example.txt", "w");
if (file_ptr == NULL) {
printf("Error opening file.\n");
} else {
printf("File opened successfully.\n");
fclose(file_ptr); // Close the file when done
}
In this example, the file example.txt
is opened in write mode. If the file is opened successfully, a success message is printed; otherwise, an error message is displayed.
Writing to a File: fprintf()
and fputc()
To write data to a file, you can use the fprintf()
function for formatted text output or the fputc()
function for writing individual characters.
Example of fprintf()
:
FILE* file_ptr = fopen("example.txt", "w");
if (file_ptr != NULL) {
fprintf(file_ptr, "Name: %s\nAge: %d\n", "John Doe", 30); // Write formatted data to the file
fclose(file_ptr); // Close the file when done
}
In this example, fprintf()
writes formatted data to the file example.txt
, including a name and age.
Example of fputc()
:
FILE* file_ptr = fopen("example.txt", "w");
if (file_ptr != NULL) {
fputc('H', file_ptr); // Write a single character to the file
fputc('i', file_ptr);
fclose(file_ptr); // Close the file when done
}
In this example, individual characters are written to the file using fputc()
.
Reading from a File: fscanf()
and fgetc()
To read data from a file, you can use the fscanf()
function for formatted input or the fgetc()
function for reading individual characters.
Example of fscanf()
:
FILE* file_ptr = fopen("example.txt", "r");
char name[50];
int age;
if (file_ptr != NULL) {
fscanf(file_ptr, "Name: %s\nAge: %d\n", name, &age); // Read formatted data from the file
printf("Name: %s, Age: %d\n", name, age);
fclose(file_ptr); // Close the file when done
}
In this example, fscanf()
reads a name and age from the file example.txt
and prints them to the console.
Example of fgetc()
:
FILE* file_ptr = fopen("example.txt", "r");
if (file_ptr != NULL) {
char ch;
while ((ch = fgetc(file_ptr)) != EOF) { // Read one character at a time
printf("%c", ch);
}
fclose(file_ptr); // Close the file when done
}
In this example, fgetc()
reads the file character by character until the end of the file is reached.
Closing a File: fclose()
After performing operations on a file, it is important to close it using the fclose()
function. Closing a file ensures that all data is properly written to the file and frees up resources associated with the file.
Syntax:
int fclose(FILE* file_ptr);
Example:
FILE* file_ptr = fopen("example.txt", "r");
if (file_ptr != NULL) {
// Perform file operations
fclose(file_ptr); // Close the file when done
}
In this example, the file is opened for reading, and fclose()
is used to close the file after operations are complete.
Error Handling in File Operations
When working with files, error handling is essential to ensure that the program behaves correctly even if file operations fail. Always check the return value of functions like fopen()
, fscanf()
, and fgetc()
to detect errors and handle them appropriately.
Example of Error Handling:
FILE* file_ptr = fopen("non_existent_file.txt", "r");
if (file_ptr == NULL) {
perror("Error opening file"); // Print the error message
} else {
// Perform file operations
fclose(file_ptr);
}
In this example, perror()
prints an error message if the file cannot be opened.
Conclusion
File handling in C enables programs to store, retrieve, and manipulate data in files, providing persistent storage for data beyond the lifetime of the program. By using functions such as fopen()
, fprintf()
, fscanf()
, fputc()
, and fgetc()
, you can efficiently read from and write to files. Properly managing file resources by closing files with fclose()
and implementing error handling are key to writing robust file-handling code in C.
Introduction to Data Structures in C: Organizing and Managing Data Efficiently
Data structures are fundamental concepts in computer science that allow programmers to organize, manage, and store data efficiently. In C programming, data structures play a vital role in solving complex problems and optimizing the performance of applications. Understanding and using data structures is essential for writing efficient and effective C programs. In this article, we will introduce the concept of data structures, explore the common types of data structures in C, and discuss their importance in programming.
What is a Data Structure?
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently. Data structures provide a means to manage large amounts of data, enabling operations such as searching, sorting, insertion, deletion, and traversal. Choosing the right data structure for a particular problem can significantly improve the efficiency of an algorithm.
In C, data structures are implemented using variables, arrays, pointers, and structures (structs). Each type of data structure serves a different purpose and is suited for specific types of tasks.
Why Use Data Structures?
Data structures are crucial for several reasons:
- Efficiency: Data structures enable efficient storage, retrieval, and manipulation of data. For example, an array allows for fast access to elements by index, while a linked list enables easy insertion and deletion of elements.
- Memory Management: Data structures help manage memory effectively by organizing data in a way that minimizes waste and optimizes resource usage.
- Problem Solving: Certain problems require specific data structures for optimal solutions. For example, a stack is ideal for problems involving depth-first search, while a queue is well-suited for breadth-first search.
- Scalability: Proper use of data structures allows programs to handle larger datasets and scale effectively as the amount of data grows.
Common Data Structures in C
In C programming, several basic and advanced data structures are commonly used to solve different types of problems. Below is an overview of some of the most widely used data structures:
- Arrays: An array is a collection of elements of the same type, stored in contiguous memory locations. Arrays allow fast access to elements using their index, making them useful for problems involving static collections of data. However, arrays have fixed sizes and do not allow for dynamic resizing.
- Linked Lists: A linked list is a linear data structure in which elements (nodes) are linked using pointers. Each node contains a data element and a pointer to the next node in the list. Linked lists are dynamic and allow efficient insertion and deletion of elements, but accessing elements by index is slower compared to arrays.
- Stacks: A stack is a last-in, first-out (LIFO) data structure that allows insertion and deletion of elements from only one end (the top). Stacks are commonly used in algorithms involving recursion, backtracking, and depth-first search.
- Queues: A queue is a first-in, first-out (FIFO) data structure in which elements are added at the rear and removed from the front. Queues are useful for handling tasks in the order they arrive, such as in scheduling algorithms or breadth-first search.
- Trees: A tree is a hierarchical data structure consisting of nodes connected by edges. The top node is called the root, and each node can have child nodes. Trees are useful for representing hierarchical relationships and are commonly used in algorithms like searching (binary search trees) and sorting (heaps).
- Binary Trees: A binary tree is a tree in which each node has at most two children, referred to as the left child and the right child. Binary trees are the foundation for binary search trees, heaps, and AVL trees, which are essential for efficient searching, sorting, and balancing operations.
- Graphs: A graph is a collection of nodes (vertices) connected by edges. Graphs can be used to represent networks, such as social networks or transportation routes. Graph algorithms are used for tasks like finding the shortest path, detecting cycles, and exploring connected components.
Choosing the Right Data Structure
The choice of data structure depends on the specific requirements of the problem at hand. Here are some factors to consider when choosing a data structure:
- Access Speed: If fast access to elements is a priority, an array may be the best choice. However, if you need to frequently insert or delete elements, a linked list might be more suitable.
- Memory Usage: Consider the memory requirements of the data structure. For example, arrays allocate a fixed amount of memory, while linked lists dynamically allocate memory as needed.
- Data Relationships: For hierarchical data, trees are often the best choice, while graphs are ideal for representing complex networks of relationships.
- Operations: Choose the data structure that efficiently supports the operations required for the problem, such as searching, sorting, insertion, and deletion.
Conclusion
Data structures are essential building blocks in C programming, providing the foundation for organizing and managing data efficiently. Understanding the various data structures available in C, such as arrays, linked lists, stacks, queues, trees, and graphs, allows programmers to select the most appropriate structure for their specific needs. By mastering data structures, you can write more efficient, scalable, and maintainable programs that effectively solve complex problems.
Linked Lists in C: Dynamic Data Structures
Linked lists are dynamic data structures that allow for efficient insertion and deletion of elements. Unlike arrays, linked lists do not have a fixed size, and their elements (nodes) are stored non-contiguously in memory. Linked lists are a fundamental data structure in C, used in a variety of applications, such as managing dynamic memory, implementing stacks, queues, and graphs. In this article, we will explore the concept of linked lists in C, including how to define, create, and manipulate them.
What is a Linked List?
A linked list is a linear data structure in which elements, called nodes, are linked using pointers. Each node contains two parts: the data to be stored and a pointer that links to the next node in the sequence. The last node in a linked list points to NULL
, indicating the end of the list.
There are several types of linked lists, but the most common are:
- Singly Linked List: Each node points to the next node in the sequence, and the last node points to
NULL
. - Doubly Linked List: Each node has two pointers: one pointing to the next node and one pointing to the previous node.
- Circular Linked List: The last node in the list points back to the first node, forming a circular structure.
Structure of a Node
In C, a node in a linked list is represented as a structure. A typical node structure for a singly linked list looks like this:
struct Node {
int data; // Data stored in the node
struct Node* next; // Pointer to the next node
};
Here, data
holds the value of the node, and next
is a pointer to the next node in the list. The type struct Node*
refers to the pointer that will hold the address of the next node.
Creating a Linked List
To create a linked list, you need to dynamically allocate memory for the nodes and link them together. Let’s start by creating a simple singly linked list with three nodes.
Example:
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
// Allocate memory for nodes in the linked list
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
// Assign data to nodes and link them
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
return 0;
}
In this example, three nodes are created and linked together to form a simple linked list with the elements 1, 2, and 3. Memory is dynamically allocated using the malloc()
function, and the nodes are linked by assigning the next
pointers.
Traversing a Linked List
To access or display the elements of a linked list, you need to traverse the list from the head node to the last node, following the next
pointers. Let’s see how to traverse a linked list and print its elements:
void printList(struct Node* n) {
while (n != NULL) {
printf("%d -> ", n->data);
n = n->next;
}
printf("NULL\n");
}
int main() {
// (Code for creating a linked list goes here)
printList(head); // Call the function to print the list
return 0;
}
In this example, the printList()
function takes a pointer to the head node and prints the data of each node until it reaches NULL
, indicating the end of the list.
Inserting Nodes in a Linked List
Inserting new nodes into a linked list can be done in several ways, such as inserting at the beginning, at the end, or after a specific node. Let’s look at how to insert a new node at the beginning of a linked list.
Inserting at the Beginning
void insertAtBeginning(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = *head_ref; // Link the new node to the current head
*head_ref = new_node; // Move the head to point to the new node
}
int main() {
struct Node* head = NULL;
// Insert new nodes at the beginning
insertAtBeginning(&head, 1);
insertAtBeginning(&head, 2);
insertAtBeginning(&head, 3);
printList(head); // Print the updated list
return 0;
}
In this example, a new node is inserted at the beginning of the list by updating the head pointer to point to the new node. The function takes a double pointer to the head to modify the head within the function.
Deleting Nodes in a Linked List
To delete a node from a linked list, you must adjust the pointers to remove the node from the chain without breaking the list. Let’s see how to delete a node given its key (the data it holds).
Deleting a Node by Key
void deleteNode(struct Node** head_ref, int key) {
struct Node* temp = *head_ref;
struct Node* prev = NULL;
// If the head node holds the key
if (temp != NULL && temp->data == key) {
*head_ref = temp->next; // Change head
free(temp); // Free old head
return;
}
// Search for the key and keep track of the previous node
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
// If the key was not present in the list
if (temp == NULL) return;
prev->next = temp->next; // Unlink the node from the list
free(temp); // Free the memory of the deleted node
}
int main() {
// (Code for creating a linked list goes here)
deleteNode(&head, 2); // Delete node with data = 2
printList(head); // Print the updated list
return 0;
}
This function deletes a node with a specific key by first locating it, then updating the pointers to bypass the node, and finally freeing the node's memory.
Advantages of Linked Lists
- Dynamic Size: Linked lists do not have a fixed size, allowing for dynamic memory allocation and efficient use of memory.
- Efficient Insertion/Deletion: Linked lists allow for fast insertion and deletion of elements, especially at the beginning or end, without shifting elements like in arrays.
- Flexible Memory Allocation: Linked lists can grow and shrink in size as needed during program execution.
Disadvantages of Linked Lists
- Memory Overhead: Linked lists require extra memory for the pointer field in each node.
- Sequential Access: Linked lists do not support random access like arrays, meaning that accessing an element requires traversing the list from the head.
- Complexity: Manipulating linked lists (insertion, deletion) is more complex and error-prone compared to arrays.
Conclusion
Linked lists are a powerful data structure in C that offer flexibility and efficiency in memory management. Understanding how to create, traverse, insert, and delete nodes in a linked list is essential for solving various programming problems. Although linked lists may introduce complexity, their dynamic nature and efficient use of memory make them a valuable tool in situations where arrays fall short.
Stacks and Queues in C: Managing Data with Linear Structures
Stacks and queues are linear data structures used to manage data efficiently in C programming. Both data structures allow for systematic data insertion and removal, but they differ in their approach. Stacks follow the Last In, First Out (LIFO) principle, while queues follow the First In, First Out (FIFO) principle. These structures are commonly used in algorithms such as expression evaluation, backtracking, scheduling, and more. In this article, we will explore stacks and queues, how to implement them in C, and their practical applications.
What is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. In a stack, the last element added to the stack is the first one to be removed. The basic operations associated with stacks are push()
(to insert an element), pop()
(to remove the top element), and peek()
(to view the top element without removing it).
Think of a stack as a stack of plates—only the top plate can be removed or added.
Stack Operations
push()
: Adds an element to the top of the stack.pop()
: Removes the top element from the stack.peek()
: Returns the top element without removing it.isEmpty()
: Checks if the stack is empty.isFull()
: Checks if the stack is full (in case of fixed-size implementations).
Implementing a Stack in C
A stack can be implemented using arrays or linked lists. Here, we will implement a stack using an array.
#include <stdio.h>
#define MAX 5 // Maximum size of the stack
int stack[MAX];
int top = -1; // Initialize stack as empty
// Function to push an element onto the stack
void push(int data) {
if (top == MAX - 1) {
printf("Stack overflow\n");
} else {
top++;
stack[top] = data;
printf("%d pushed onto the stack\n", data);
}
}
// Function to pop an element from the stack
int pop() {
if (top == -1) {
printf("Stack underflow\n");
return -1;
} else {
int popped = stack[top];
top--;
printf("%d popped from the stack\n", popped);
return popped;
}
}
// Function to peek at the top element
int peek() {
if (top == -1) {
printf("Stack is empty\n");
return -1;
} else {
return stack[top];
}
}
int main() {
push(10);
push(20);
push(30);
printf("Top element is %d\n", peek());
pop();
printf("Top element is %d\n", peek());
return 0;
}
In this example, we define a stack of integers with a fixed size of 5. The push()
, pop()
, and peek()
operations are implemented, allowing you to interact with the stack.
What is a Queue?
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. In a queue, the first element added is the first one to be removed. The basic operations associated with queues are enqueue()
(to insert an element), dequeue()
(to remove the front element), and peek()
(to view the front element without removing it).
Think of a queue as a line of people waiting for service—the first person in line is the first to be served.
Queue Operations
enqueue()
: Adds an element to the rear of the queue.dequeue()
: Removes the front element from the queue.peek()
: Returns the front element without removing it.isEmpty()
: Checks if the queue is empty.isFull()
: Checks if the queue is full (in case of fixed-size implementations).
Implementing a Queue in C
A queue can be implemented using arrays or linked lists. Here, we will implement a queue using an array.
#include <stdio.h>
#define MAX 5 // Maximum size of the queue
int queue[MAX];
int front = -1, rear = -1;
// Function to enqueue an element into the queue
void enqueue(int data) {
if (rear == MAX - 1) {
printf("Queue overflow\n");
} else {
if (front == -1) front = 0;
rear++;
queue[rear] = data;
printf("%d enqueued into the queue\n", data);
}
}
// Function to dequeue an element from the queue
int dequeue() {
if (front == -1 || front > rear) {
printf("Queue underflow\n");
return -1;
} else {
int dequeued = queue[front];
front++;
printf("%d dequeued from the queue\n", dequeued);
return dequeued;
}
}
// Function to peek at the front element
int peek() {
if (front == -1 || front > rear) {
printf("Queue is empty\n");
return -1;
} else {
return queue[front];
}
}
int main() {
enqueue(10);
enqueue(20);
enqueue(30);
printf("Front element is %d\n", peek());
dequeue();
printf("Front element is %d\n", peek());
return 0;
}
In this example, we define a queue of integers with a fixed size of 5. The enqueue()
, dequeue()
, and peek()
operations are implemented, allowing you to interact with the queue.
Applications of Stacks and Queues
- Stacks: Stacks are used in applications such as expression evaluation (e.g., converting infix to postfix expressions), backtracking algorithms (e.g., solving mazes), and managing function calls in recursive programming (e.g., the call stack).
- Queues: Queues are used in applications such as scheduling algorithms (e.g., round-robin scheduling), managing tasks in breadth-first search (BFS) algorithms, and handling data streams (e.g., printers, CPU task scheduling).
Conclusion
Stacks and queues are fundamental data structures that provide efficient ways to manage data in linear order. Stacks follow the Last In, First Out (LIFO) principle, making them ideal for tasks such as function call management and backtracking, while queues follow the First In, First Out (FIFO) principle, making them suitable for scheduling and managing tasks in sequential order. By understanding how to implement and use stacks and queues in C, you can solve a wide range of problems effectively.
Trees and Binary Trees in C: Hierarchical Data Structures
Trees are hierarchical data structures that consist of nodes connected by edges. Unlike linear data structures like arrays, stacks, and queues, trees are non-linear and can represent hierarchical relationships. A binary tree is a special type of tree where each node has at most two children, referred to as the left child and the right child. Trees and binary trees are widely used in areas such as expression parsing, searching algorithms, and representing hierarchical data like file systems. In this article, we will explore the concepts of trees and binary trees, how to implement them in C, and their practical applications.
What is a Tree?
A tree is a collection of nodes organized in a hierarchical structure. Each node contains data, and nodes are connected by edges that define the parent-child relationship. The topmost node in a tree is called the root, and every node in the tree, except the root, has exactly one parent. Nodes with no children are called leaf nodes.
Properties of Trees:
- Root: The topmost node in the tree.
- Parent: A node that has children connected to it.
- Child: A node that descends from another node (parent).
- Leaf: A node that has no children.
- Height: The number of edges on the longest path from the root to a leaf.
What is a Binary Tree?
A binary tree is a special type of tree where each node has at most two children: a left child and a right child. Binary trees are commonly used in search algorithms, sorting algorithms (e.g., binary search trees), and expression evaluation.
Types of Binary Trees:
- Full Binary Tree: Every node has either 0 or 2 children.
- Complete Binary Tree: All levels are completely filled except possibly the last level, which is filled from left to right.
- Perfect Binary Tree: All internal nodes have two children, and all leaf nodes are at the same level.
- Balanced Binary Tree: The height difference between the left and right subtrees of every node is at most 1.
- Binary Search Tree (BST): A binary tree where for every node, the value of the left child is less than the parent, and the value of the right child is greater than the parent.
Implementing a Binary Tree in C
To implement a binary tree in C, we define a structure for the tree node. Each node contains data and pointers to the left and right children.
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a tree node
struct Node {
int data;
struct Node* left;
struct Node* right;
};
// Function to create a new node
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
// Function for inorder traversal (left, root, right)
void inorderTraversal(struct Node* root) {
if (root == NULL) {
return;
}
inorderTraversal(root->left);
printf("%d ", root->data);
inorderTraversal(root->right);
}
int main() {
// Create the root node
struct Node* root = createNode(1);
// Create the left and right children
root->left = createNode(2);
root->right = createNode(3);
// Further expand the tree
root->left->left = createNode(4);
root->left->right = createNode(5);
// Perform an inorder traversal of the binary tree
printf("Inorder Traversal: ");
inorderTraversal(root);
return 0;
}
In this example, we define a binary tree with five nodes and perform an inorder traversal, which prints the nodes in the order: left subtree, root, right subtree.
Traversing a Binary Tree
Tree traversal refers to the process of visiting all the nodes in a tree in a specific order. There are several types of binary tree traversals:
- Inorder Traversal (Left, Root, Right): Visit the left subtree, the root node, and then the right subtree.
- Preorder Traversal (Root, Left, Right): Visit the root node first, then the left subtree, and finally the right subtree.
- Postorder Traversal (Left, Right, Root): Visit the left subtree, the right subtree, and then the root node.
- Level-order Traversal: Visit nodes level by level, starting from the root and moving downwards.
Example of Preorder Traversal:
void preorderTraversal(struct Node* root) {
if (root == NULL) {
return;
}
printf("%d ", root->data);
preorderTraversal(root->left);
preorderTraversal(root->right);
}
In this example, preorderTraversal()
visits the root node first, followed by the left and right subtrees.
Binary Search Tree (BST)
A Binary Search Tree (BST) is a special type of binary tree where the left child of a node contains a value less than the node’s value, and the right child contains a value greater than the node’s value. This property makes BSTs highly efficient for searching, inserting, and deleting elements.
Operations on a BST
- Insertion: Insert a new node into the BST, maintaining the BST property.
- Searching: Search for a given value by traversing the tree based on comparisons.
- Deletion: Remove a node from the BST while maintaining the BST property.
Example of Insertion in a BST:
struct Node* insert(struct Node* node, int data) {
if (node == NULL) {
return createNode(data);
}
if (data < node->data) {
node->left = insert(node->left, data);
} else if (data > node->data) {
node->right = insert(node->right, data);
}
return node;
}
int main() {
struct Node* root = NULL;
root = insert(root, 50);
insert(root, 30);
insert(root, 20);
insert(root, 40);
insert(root, 70);
insert(root, 60);
insert(root, 80);
return 0;
}
In this example, we insert nodes into a BST while maintaining the order property, which ensures that smaller values go to the left and larger values to the right.
Applications of Trees and Binary Trees
- Expression Trees: Used in compilers to evaluate arithmetic expressions.
- Binary Search Trees: Used for efficient searching, insertion, and deletion of elements.
- Decision Trees: Used in machine learning and decision-making processes.
- Heaps: A special type of binary tree used in priority queues and heap sort algorithms.
- File Systems: Trees are used to represent hierarchical file structures.
Conclusion
Trees and binary trees are fundamental data structures used to represent hierarchical data efficiently. Binary trees, in particular, are widely used in searching and sorting algorithms due to their structured nature. By understanding how to implement and manipulate trees and binary trees in C, you can solve complex problems involving hierarchical data and improve the efficiency of your algorithms.
Graphs in C: Modeling Complex Relationships
Graphs are powerful non-linear data structures that consist of a set of vertices (nodes) connected by edges (links). They are used to model relationships between different entities in various domains, such as social networks, communication networks, and transportation systems. Unlike trees, graphs can have cycles, and the connections between vertices do not follow a strict parent-child relationship. In this article, we will explore the concept of graphs, how to represent them in C, and their practical applications.
What is a Graph?
A graph is a collection of vertices connected by edges. Vertices represent the entities or objects, while edges represent the relationships between them. Graphs are versatile structures that can represent both directed and undirected relationships, and they can have weighted or unweighted edges depending on the application.
Properties of Graphs:
- Vertices (Nodes): The fundamental units or points in a graph.
- Edges (Links): The connections between vertices that define the relationships.
- Directed Graph (Digraph): A graph where edges have a direction, indicating a one-way relationship.
- Undirected Graph: A graph where edges have no direction, indicating a two-way relationship.
- Weighted Graph: A graph where edges carry weights, often used to represent costs, distances, or other attributes.
- Unweighted Graph: A graph where edges have no associated weight.
- Cyclic Graph: A graph that contains at least one cycle (a path where the first and last vertices are the same).
- Acyclic Graph: A graph without any cycles.
Representing Graphs in C
There are two common ways to represent graphs in C: using an adjacency matrix or an adjacency list.
Adjacency Matrix: This representation uses a 2D array to indicate whether there is an edge between a pair of vertices. This is more suitable for dense graphs where the number of edges is close to the number of possible edges.
Adjacency List: This representation uses an array of linked lists, where each vertex has a list of its neighboring vertices. This is more space-efficient for sparse graphs.
Types of Graphs
Graphs can be categorized into different types based on their characteristics and structure:
- Directed Graph (Digraph): A graph where each edge has a direction. In a directed graph, the relationship is one-way.
- Undirected Graph: A graph where edges have no direction, representing a bidirectional relationship between vertices.
- Weighted Graph: A graph where each edge has a weight associated with it, representing distances, costs, or other metrics.
- Unweighted Graph: A graph where edges have no associated weights, representing basic connections.
- Cyclic Graph: A graph that contains at least one cycle, meaning you can start at a vertex, follow a sequence of edges, and return to the same vertex.
- Acyclic Graph: A graph with no cycles. A common example is a tree, which is an acyclic graph.
- Connected Graph: A graph where there is a path between every pair of vertices.
- Disconnected Graph: A graph where at least one pair of vertices is not connected by any path.
Graph Traversal Algorithms
Graph traversal refers to the process of visiting all vertices in a graph. There are two primary traversal algorithms:
- Breadth-First Search (BFS): An algorithm that visits all vertices at the present depth level before moving on to the vertices at the next depth level.
- Depth-First Search (DFS): An algorithm that explores as far as possible along a branch before backtracking to explore the remaining vertices.
Both BFS and DFS are used in various graph-based problems, such as searching for a path, finding connected components, and solving mazes.
Applications of Graphs
Graphs are widely used in many fields and applications:
- Social Networks: Graphs represent connections between individuals (vertices) and their relationships (edges).
- Communication Networks: Graphs model communication systems like the Internet or telephone networks, where vertices represent routers or devices, and edges represent connections.
- Transportation Networks: Graphs are used to represent roads, railways, or flight paths, with vertices representing locations and edges representing routes.
- Search Engines: Web pages are modeled as vertices, and links between them as edges, which allows search engines to index and rank pages.
- Optimization Problems: Graphs are used to solve problems like the shortest path, minimum spanning tree, and network flow.
Conclusion
Graphs are highly versatile data structures that allow us to model and solve complex problems involving relationships between entities. Understanding how to represent, traverse, and manipulate graphs in C is essential for applications ranging from social networks to optimization algorithms. Whether using adjacency matrices or adjacency lists, graphs offer powerful tools for efficient data management and problem solving.
Sorting Algorithms in C: Organizing Data Efficiently
Sorting algorithms are fundamental techniques used to rearrange the elements of an array or list in a particular order, typically ascending or descending. Sorting is a crucial operation in many applications, from searching and data analysis to optimization problems. In this article, we will explore some of the most commonly used sorting algorithms: Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, and Quick Sort. Each algorithm has its own advantages and disadvantages, depending on the size and nature of the dataset.
What is Sorting?
Sorting refers to the process of arranging data in a specific order. Sorting algorithms are classified based on factors such as their time complexity, space complexity, and whether they are stable (i.e., preserve the order of equal elements).
Sorting algorithms can be categorized into two types:
- Comparison-based Sorting: Algorithms that compare elements to determine their order (e.g., Bubble Sort, Merge Sort, Quick Sort).
- Non-comparison Sorting: Algorithms that do not directly compare elements but use other properties of the data (e.g., Radix Sort, Counting Sort).
Bubble Sort
Bubble Sort is a simple comparison-based sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The algorithm continues this process until the list is sorted. It is called Bubble Sort because the smaller elements "bubble" to the top of the list.
Time Complexity:
- Best Case: O(n) (when the array is already sorted)
- Average Case: O(n²)
- Worst Case: O(n²)
Example of Bubble Sort in C:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap the elements
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
Selection Sort
Selection Sort is another simple comparison-based algorithm that works by repeatedly selecting the smallest (or largest) element from the unsorted part of the array and placing it at the beginning. The algorithm continues to select and place elements until the entire array is sorted.
Time Complexity:
- Best Case: O(n²)
- Average Case: O(n²)
- Worst Case: O(n²)
Example of Selection Sort in C:
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int min_idx = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[min_idx]) {
min_idx = j;
}
}
// Swap the found minimum element with the first element
int temp = arr[min_idx];
arr[min_idx] = arr[i];
arr[i] = temp;
}
}
Insertion Sort
Insertion Sort is a comparison-based algorithm that builds the sorted array one element at a time by comparing each new element with the already sorted part and inserting it in the correct position. It is efficient for small datasets and is often used when the array is nearly sorted.
Time Complexity:
- Best Case: O(n) (when the array is already sorted)
- Average Case: O(n²)
- Worst Case: O(n²)
Example of Insertion Sort in C:
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
// Move elements that are greater than key to one position ahead of their current position
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
Merge Sort
Merge Sort is a divide-and-conquer algorithm that divides the array into two halves, recursively sorts each half, and then merges the two sorted halves back together. Merge Sort is stable and has a predictable time complexity, making it efficient for large datasets.
Time Complexity:
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n log n)
Example of Merge Sort in C:
void merge(int arr[], int l, int m, int r) {
int n1 = m - l + 1;
int n2 = r - m;
// Create temp arrays
int L[n1], R[n2];
// Copy data to temp arrays L[] and R[]
for (int i = 0; i < n1; i++) {
L[i] = arr[l + i];
}
for (int i = 0; i < n2; i++) {
R[i] = arr[m + 1 + i];
}
// Merge the temp arrays back into arr[l..r]
int i = 0, j = 0, k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
// Copy the remaining elements of L[], if any
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
// Copy the remaining elements of R[], if any
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(int arr[], int l, int r) {
if (l < r) {
int m = l + (r - l) / 2;
// Sort first and second halves
mergeSort(arr, l, m);
mergeSort(arr, m + 1, r);
merge(arr, l, m, r);
}
}
Quick Sort
Quick Sort is another divide-and-conquer algorithm that selects a pivot element, partitions the array into two parts such that all elements less than the pivot are on the left and all elements greater than the pivot are on the right, and then recursively sorts the sub-arrays. Quick Sort is efficient for large datasets and is often faster than Merge Sort in practice.
Time Complexity:
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n²) (when the pivot selection is poor)
Example of Quick Sort in C:
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // Choose the last element as the pivot
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
// Recursively sort the partitions
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Conclusion
Sorting algorithms are essential for efficiently organizing data in applications ranging from searching to data analysis. Each algorithm has its own strengths and weaknesses, and the choice of algorithm depends on the size of the dataset, the nature of the data, and the required performance. Whether you are dealing with small arrays or large datasets, understanding the various sorting algorithms in C—such as Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, and Quick Sort—can help you choose the most appropriate method for your needs.
Searching Algorithms in C: Efficient Data Retrieval
Searching algorithms are fundamental techniques used to find the position of an element in a collection of data, such as an array or list. Efficient searching is crucial in computer science for applications such as databases, file systems, and data analysis. In this article, we will explore two commonly used searching algorithms: Linear Search and Binary Search. Each algorithm has its own advantages and is used depending on the nature of the data being searched.
What is Searching?
Searching is the process of finding a particular element in a collection of data. The goal is to determine whether the element exists in the dataset, and if so, to return its position. Searching algorithms can be categorized based on their approach and efficiency.
Types of Searching Algorithms:
- Linear Search: A simple algorithm that checks each element of the list sequentially until the desired element is found or the list ends.
- Binary Search: A more efficient algorithm that divides the search space in half with each step, but requires the data to be sorted beforehand.
Linear Search
Linear Search is the simplest searching algorithm. It works by checking each element in the list sequentially until the target element is found or the list is exhausted. This algorithm does not require the list to be sorted, making it a versatile option for unsorted datasets.
Time Complexity:
- Best Case: O(1) (when the target element is the first element in the list)
- Average Case: O(n)
- Worst Case: O(n) (when the target element is the last element or not present)
Example of Linear Search in C:
int linearSearch(int arr[], int n, int x) {
for (int i = 0; i < n; i++) {
if (arr[i] == x) {
return i; // Return the index of the found element
}
}
return -1; // Element not found
}
int main() {
int arr[] = {10, 20, 30, 40, 50};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 30;
int result = linearSearch(arr, n, x);
if (result == -1) {
printf("Element not found\n");
} else {
printf("Element found at index %d\n", result);
}
return 0;
}
In this example, Linear Search is used to find the element 30
in an unsorted array. The algorithm checks each element sequentially until the target is found or the search ends.
Binary Search
Binary Search is a more efficient searching algorithm that works by repeatedly dividing the search interval in half. The algorithm requires the dataset to be sorted beforehand. It compares the middle element of the array with the target value. If the target is equal to the middle element, the search is complete. If the target is smaller, the algorithm continues searching the left half, and if the target is larger, the search continues in the right half.
Time Complexity:
- Best Case: O(1) (when the target element is the middle element)
- Average Case: O(log n)
- Worst Case: O(log n)
Example of Binary Search in C:
int binarySearch(int arr[], int l, int r, int x) {
while (l <= r) {
int m = l + (r - l) / 2;
// Check if x is present at mid
if (arr[m] == x) {
return m;
}
// If x is greater, ignore the left half
if (arr[m] < x) {
l = m + 1;
}
// If x is smaller, ignore the right half
else {
r = m - 1;
}
}
return -1; // Element not found
}
int main() {
int arr[] = {10, 20, 30, 40, 50};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 30;
int result = binarySearch(arr, 0, n - 1, x);
if (result == -1) {
printf("Element not found\n");
} else {
printf("Element found at index %d\n", result);
}
return 0;
}
In this example, Binary Search is used to find the element 30
in a sorted array. The algorithm divides the array into halves and efficiently narrows down the search space until the target element is found.
Comparison of Linear Search and Binary Search
The choice between Linear Search and Binary Search depends on the nature of the dataset and the efficiency required:
- Linear Search: Can be used on unsorted datasets but is less efficient for large datasets due to its O(n) time complexity.
- Binary Search: Requires the dataset to be sorted but offers much better performance with a time complexity of O(log n). It is ideal for large datasets where sorting is feasible.
Applications of Searching Algorithms
- Databases: Searching algorithms are used to quickly retrieve records in large databases.
- File Systems: Searching is essential for finding files and directories in hierarchical file systems.
- Data Analysis: Searching is used to locate specific data points within large datasets during analysis.
- Text Processing: Searching algorithms are used in text editors and word processors to locate specific words or phrases within a document.
Conclusion
Searching algorithms are essential for efficient data retrieval in a wide range of applications. Linear Search provides a straightforward approach for unsorted datasets, while Binary Search offers a more efficient solution for sorted datasets. By understanding the strengths and weaknesses of each algorithm, you can choose the most appropriate method for your specific data retrieval needs.
Recursion in C: Solving Problems by Breaking Them Down
Recursion is a powerful programming technique where a function calls itself to solve smaller instances of a problem. It is particularly useful for problems that can be naturally divided into similar subproblems, such as in mathematical computations, algorithm design, and tree or graph traversal. Recursion allows for elegant solutions to problems that may be difficult to solve iteratively. In this article, we will explore the concept of recursion in C, understand how it works, and examine some common applications.
What is Recursion?
Recursion occurs when a function calls itself directly or indirectly. Each recursive call works on a smaller instance of the original problem, and eventually, the function reaches a base case that does not involve any further recursive calls. This base case prevents infinite recursion and allows the function to return and resolve the larger problem step by step.
Every recursive function must have two key components:
- Base Case: The condition that stops the recursion and prevents infinite function calls.
- Recursive Case: The part of the function that calls itself to solve a smaller version of the original problem.
How Recursion Works
When a recursive function is called, the program keeps track of each recursive call using a call stack. Each function call is pushed onto the stack, and when the base case is reached, the calls are popped off the stack as the function returns. This stack-based behavior is what allows recursion to work correctly, as each recursive call can rely on the state of the previous calls.
Types of Recursion
Recursion can be categorized into two types:
- Direct Recursion: A function that calls itself directly.
- Indirect Recursion: A function that calls another function, which eventually calls the original function.
Direct recursion is the most common type used in problem-solving, while indirect recursion is less common but still valuable in certain scenarios.
Example of Recursion: Factorial
One of the simplest and most common examples of recursion is calculating the factorial of a number. The factorial of a number n
is defined as the product of all positive integers from 1 to n
. It is represented as n!
.
The recursive definition of a factorial is:
- Base Case:
0! = 1
and1! = 1
- Recursive Case:
n! = n * (n - 1)!
Example of a recursive function to calculate the factorial in C:
int factorial(int n) {
if (n == 0 || n == 1) {
return 1; // Base case
} else {
return n * factorial(n - 1); // Recursive case
}
}
int main() {
int num = 5;
printf("Factorial of %d is %d\n", num, factorial(num));
return 0;
}
In this example, the function factorial()
recursively calls itself to calculate the factorial of the number. The base case ensures that the recursion terminates when n
reaches 0 or 1.
Example of Recursion: Fibonacci Sequence
The Fibonacci sequence is another common example of recursion. In this sequence, each number is the sum of the two preceding numbers, starting with 0 and 1. The recursive definition of the Fibonacci sequence is:
- Base Case:
fib(0) = 0
andfib(1) = 1
- Recursive Case:
fib(n) = fib(n - 1) + fib(n - 2)
Example of a recursive function to generate the nth Fibonacci number in C:
int fibonacci(int n) {
if (n == 0) {
return 0; // Base case
} else if (n == 1) {
return 1; // Base case
} else {
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
}
int main() {
int num = 6;
printf("Fibonacci number at position %d is %d\n", num, fibonacci(num));
return 0;
}
In this example, the function fibonacci()
recursively calls itself to calculate the nth Fibonacci number. The base cases ensure that the recursion terminates when n
is 0 or 1.
Advantages and Disadvantages of Recursion
Recursion can provide elegant solutions to problems that involve repeated patterns or can be broken down into similar subproblems. However, it also comes with trade-offs:
- Advantages:
- Simplifies the code for problems that have a natural recursive structure (e.g., tree traversal, factorial calculation).
- Reduces the need for complex loops and auxiliary data structures.
- Disadvantages:
- Recursion can be inefficient for large problems due to the overhead of multiple function calls and the risk of stack overflow.
- May lead to slower execution time for problems that can be solved more efficiently with iteration.
Applications of Recursion
Recursion is widely used in various algorithms and problem-solving techniques:
- Tree Traversal: Recursion is commonly used in traversing trees, such as in-order, pre-order, and post-order traversal of binary trees.
- Graph Traversal: Recursive algorithms like Depth-First Search (DFS) are used to explore graphs.
- Divide and Conquer Algorithms: Algorithms such as Merge Sort, Quick Sort, and Binary Search utilize recursion to break down problems into smaller subproblems.
- Dynamic Programming: Many dynamic programming problems, such as calculating Fibonacci numbers or solving the knapsack problem, are solved recursively with memoization.
- Backtracking: Recursion is used in backtracking algorithms to explore all possible solutions (e.g., solving a Sudoku puzzle).
Conclusion
Recursion is a powerful technique in C programming that simplifies the solution of complex problems by breaking them down into smaller, similar subproblems. While recursion provides elegant and concise code for problems like tree traversal and factorial computation, it comes with challenges such as potential inefficiency and the risk of stack overflow for large inputs. By understanding how to use recursion effectively, you can tackle a wide range of algorithmic problems in a more structured and efficient manner.
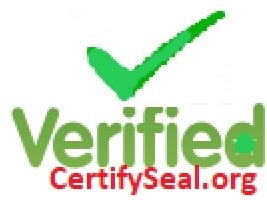